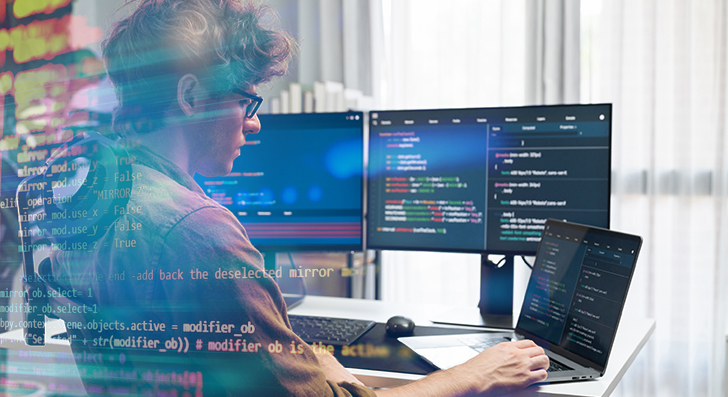
Scalability suggests your application can deal with growth—additional end users, a lot more info, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they mature quickly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your method will behave stressed.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting The complete system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 people or just a hundred? Choose the proper sort—relational or NoSQL—determined by how your facts will grow. Strategy for sharding, indexing, and backups early, even if you don’t need to have them still.
Another important issue is to avoid hardcoding assumptions. Don’t create code that only operates beneath existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that support scaling, like information queues or event-pushed units. These assistance your app deal with much more requests with out obtaining overloaded.
When you build with scalability in your mind, you are not just getting ready for success—you are lowering long term headaches. A perfectly-prepared technique is simpler to maintain, adapt, and develop. It’s better to arrange early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a crucial part of setting up scalable apps. Not all databases are crafted precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your app grows.
Get started by knowledge your info. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good in shape. They're strong with associations, transactions, and regularity. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and data.
When your data is much more adaptable—like person activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more effortlessly.
Also, look at your read and publish styles. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a significant write load? Explore databases that could tackle higher compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent info streams).
It’s also sensible to Assume in advance. You might not require Superior scaling capabilities now, but deciding on a databases that supports them means you won’t require to change afterwards.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data depending on your access patterns. And usually keep track of database overall performance while you increase.
Briefly, the appropriate databases is dependent upon your app’s construction, speed requirements, and how you anticipate it to grow. Get time to choose wisely—it’ll save a lot of hassle afterwards.
Enhance Code and Queries
Rapidly code is key to scalability. As your app grows, each individual compact delay provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s important to Establish successful logic from the start.
Start by crafting cleanse, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated solution if an easy a single works. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take much too prolonged to run or works by using a lot of memory.
Subsequent, evaluate your database queries. These normally sluggish matters down over the code alone. Ensure each query only asks for the info you actually will need. Steer clear of SELECT *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, especially across substantial tables.
In the event you observe exactly the same facts being requested time and again, use caching. Retail store the outcomes briefly applying tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your application much more effective.
Remember to take a look at with substantial datasets. Code and queries that work good with 100 documents could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more consumers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application speedy, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing all of the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing knowledge temporarily so it might be reused speedily. When consumers ask for precisely the same details again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You'll be able to provide it through the cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And often be certain your cache is up to date when facts does alter.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of extra customers, keep speedy, and recover from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Allow you to rent servers and providers as you may need them. You don’t should invest in components or guess future capability. When traffic raises, you'll be able to include far more assets with just a couple clicks or routinely employing car-scaling. When targeted visitors drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You may center on making your application more info as an alternative to handling infrastructure.
Containers are Yet another important tool. A container offers your application and every little thing it must run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for general performance and dependability.
In short, working with cloud and container resources means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when things go Improper. Checking allows you see how your app is executing, location challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently glitches transpire, and where by they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before consumers even discover.
Checking is likewise practical after you make improvements. In case you deploy a fresh function and find out a spike in problems or slowdowns, you'll be able to roll it back right before it will cause true harm.
As your application grows, targeted traffic and information increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the correct applications in position, you stay on top of things.
In a nutshell, checking will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about knowledge your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create smart.